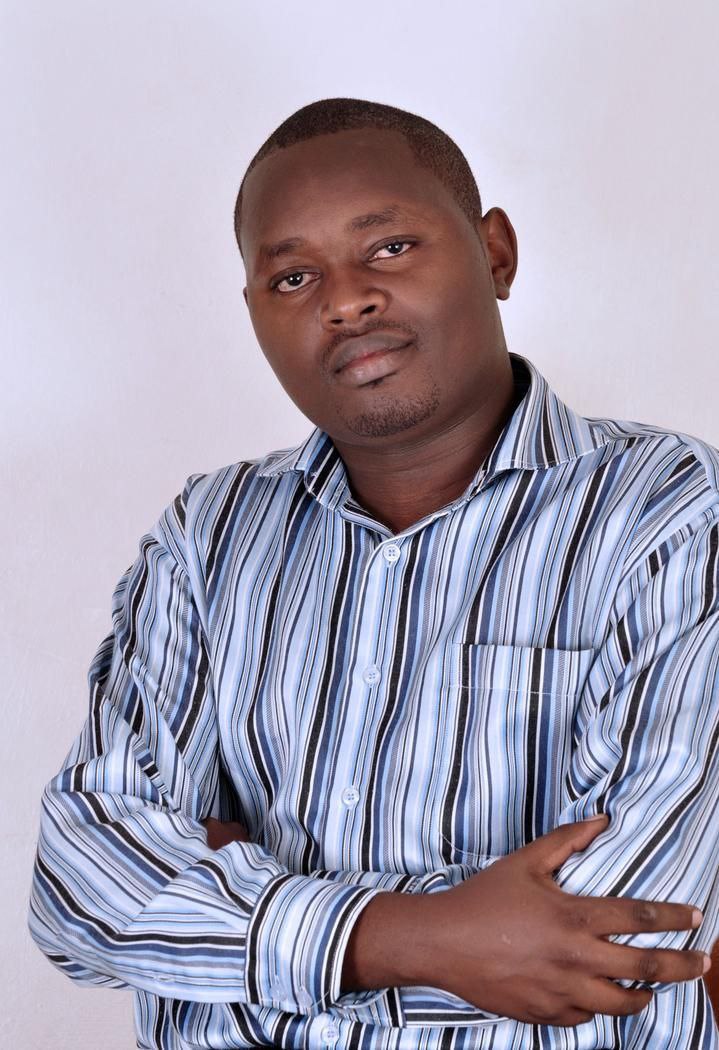
Father, Husband, Thinker, Creative
I am passionate about all things technology.
Funny faces for your apps
A Cross-Environment SVG Avatar Generator
Need a funny avatar for your apps? I do too!
There exists a number of APIS out there to help you generate avatars based on a user's email or some other unique seed. But there are moments when an external API won't work. A good example is when you are building an APP that is expected to still function effectively with or without an internet connection.
Facitars was built for you, and for those scenarios.
The Core Challenge
When building facitars, I set out to achieve these simple goals:
- Generate unique avatars for users
- Make no external API calls
- Work consistently in both browser and Node.js
- Keep the bundle size small
- Generate deterministic but random-looking results
- Include color information for UI theming
The Solution: SVG.js + Seed-Based Generation
The core insight was using SVG.js as the rendering engine, combined with seed-based randomization. Here's a basic example:
const facitars = new Facitars();
// Generate a 300x300px avatar
const { svg, color } = await facitars.generate('user@email.com', 300);
The magic happens in how we handle the environment differences:
class Facitars {
constructor(opts) {
// Browser mode - use window
if (typeof opts !== 'object') {
return;
}
// Node.js mode - set up JSDOM
const { JSDOM } = opts.jsdom;
const { window } = new JSDOM(
`<html><head><script>${opts.localSvgJs}</script></head></html>`,
{ runScripts: 'dangerously' }
);
this.window = window;
}
}
Seed-Based Feature Generation
Instead of pure randomness, each avatar feature is deterministically generated based on the input seed. This ensures the same seed always produces the same avatar:
class Facitars {
#get_seed_val(arr, type = '') {
this.count++;
let seed = this.seed + '-' + type + '-' + this.count * 10000;
let w_arr = new Array(arr.length)
.fill(0)
.map((v) => Math.ceil(100 / arr.length));
let i = chooser.chooseWeightedIndex(w_arr, seed);
return arr[i];
}
}
Building the Face
The avatar is constructed from simple SVG primitives, keeping both the code and output lightweight:
face() {
const faces_arr = [
{ shape: 'rect', args: [Size, Size] },
{ shape: 'circle', args: [Size] },
// More variations...
];
const { shape, args, radius, move } = this.#get_seed_val(faces_arr);
let face = this.draw[shape](...args);
if (radius) face.radius(radius);
if (move) face.move(...move);
face.fill(this.colorHex);
}
Each feature (eyes, nose, mouth) follows a similar pattern - select from predefined variations based on the seed.
Cross-Environment Compatibility
The trickiest part was ensuring consistent rendering between browser and server. The solution was to:
- Package a local copy of SVG.js
- For Node.js use JSDOM to provide DOM APIs server-side
- Stick to basic SVG operations that work reliably across environments
// Browser usage
const Facitar = require('facitars').browser();
const facitar = new Facitar();
// Server usage
const Facitar = require('facitars').node();
const facitar = new Facitar();
The final bundle is just 17.8KB minified, works in any JavaScript environment, and generates unique, deterministic avatars with matching colors.
Using in Production
The module is easy to integrate into both browser and Node.js applications:
// Browser
<script src="/dist/facitars.min.js"></script>
const facitars = new Facitars();
// Node.js
const Facitars = require('facitars').node();
const facitars = new Facitars();
// Both environments
const { svg, color } = await facitars.generate('unique-seed', 300);
Future Improvements
While the current version meets its core goals, there's room for enhancement:
- Additional face variations while maintaining the small bundle size
- More control over color generation
- Animation support for interactive applications
- Custom feature sets for different avatar styles
Sometimes the best solution isn't the most complex one. By focusing on simplicity, cross-environment compatibility, and deterministic generation, Facitars provides a lightweight yet powerful tool for avatar generation without external dependencies.
Happy Geeking!