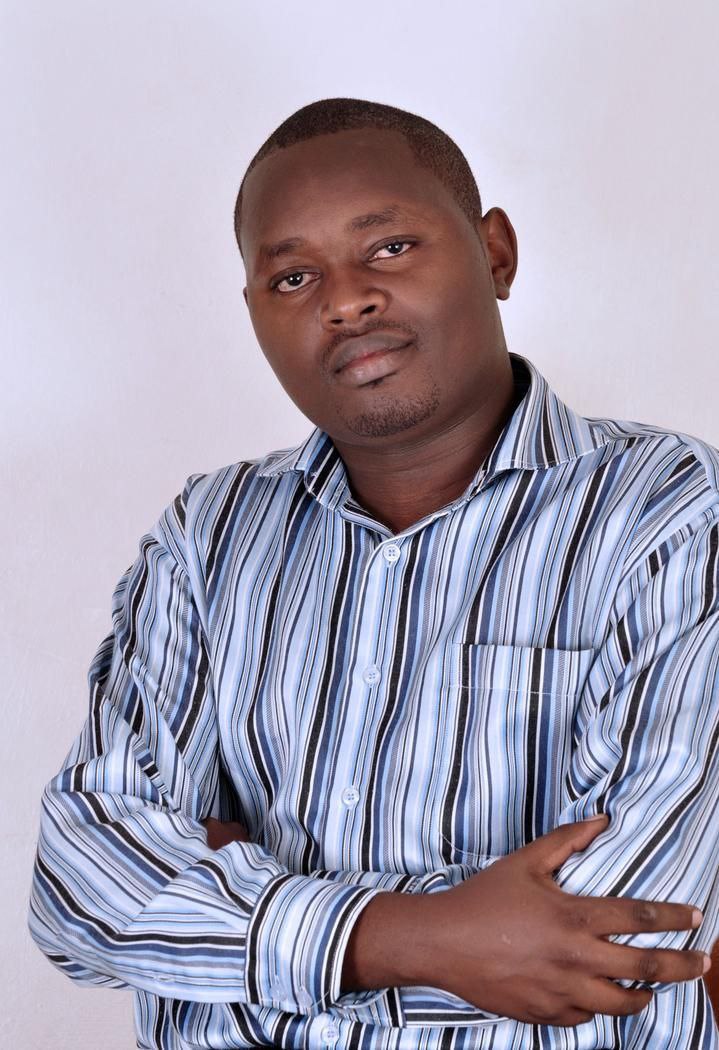
Father, Husband, Thinker, Creative
I am passionate about all things technology.
Vision-capable AI can be tricky
Working with AI image models should be straightforward. Feed in an image, get predictions back. But if you've ever integrated with multiple AI APIs, you know the reality is messier. Every API requires your image data in a slightly different format – base64 strings, binary buffers, data URLs, or streams.
After wrestling with these inconsistencies across Gemini, LLAMA, Claude, and Qwen integrations, I built file-to-url to handle the tedious bits. It's a tiny module that takes care of the conversions as well as resizes the image so that it is not too big, letting you focus on the actual AI integration.
The Core Problem
Here's what typically happens when you're integrating multiple AI image models:
- Gemini wants a base64-encoded image
- Another API needs a binary buffer
- Yet another expects a data URL
- And suddenly you're juggling different image formats, sizes, and encodings
You end up writing repetitive utility functions for each integration. Not fun.
Simple File Handling
Here's how simple it becomes:
// Load and optimize an image for AI processing
const handler = await FileHandler.handle('photo.jpg', {
maxWidth: 800,
maxHeight: 800,
quality: 90,
format: 'jpeg'
});
// Now get whatever format you need
const base64 = handler.toBase64(); // For Gemini
const buffer = handler.toBuffer(); // For binary APIs
const dataUrl = handler.toBase64URL(); // For browser-based APIs
const stream = handler.toStream(); // For streaming APIs
The Sharp Edge
Let me geek out about Sharp for a moment. After years of working with various image processing libraries, Sharp is hands down the best module on the NPM landscape. It's fast, memory-efficient, and just reliable. Also I have found it to have about every feature I ever needed for my tasks. But the best part is that it's installation is straightforward. It truly defines the meaning of 'batteries included'! This module relies on Sharp for image manipulation.
The Base64 Validation Saga
Here's a fun one: When working with mixed Python/Node.js pipelines, images sometimes arrive pre-encoded in base64. Detecting this reliably is surprisingly tricky – you can't just check if a string looks base64-ish. After some hair-pulling moments with malformed data, I added robust validation:
// Don't re-encode if it's already good to go
if (FileHandler.isBase64DataURL(imageData)) {
// Use as is
} else {
const handler = await FileHandler.handle(imageData);
const base64 = handler.toBase64URL();
}
In Practice
Here's a real-world example handling images for multiple AI services:
async function processWithMultipleAIs(imagePath) {
const handler = await FileHandler.handle(imagePath);
// For Gemini
const geminiPayload = {
inlineData: {
data: handler.toBase64(),
mimeType: handler.mimeType
}
};
// For Claude
const claudePayload = {
file: handler.toBase64URL()
};
// For custom API requiring binary
const binaryPayload = new FormData();
binaryPayload.append('file', handler.toBlob());
return {
gemini: await callGemini(geminiPayload),
claude: await callClaude(claudePayload),
custom: await callCustomAPI(binaryPayload)
};
}
What's Next?
While FileHandler already handles most common cases, there's always room for improvement:
- Adding support for more image optimization options
- Handling video files for video-capable AI models
- Adding batch processing capabilities
- Better documentation. I know 🤦, will work on it.
If you're working with AI image processing, give it a shot. And if you have suggestions or run into interesting edge cases, the GitHub repository is open for issues and contributions.
Happy Geeking!